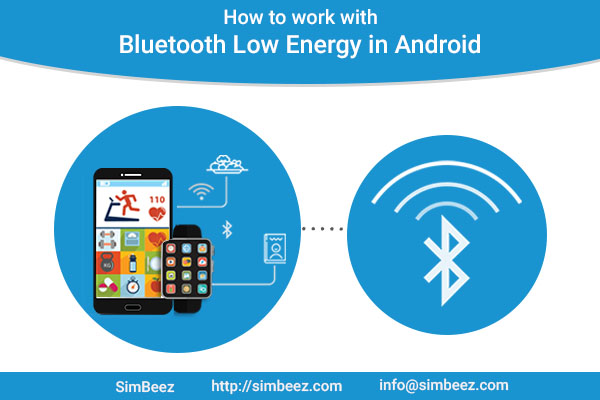
Bluetooth is one of the most used technology when the connection has to be established with a remote device. But this technology has a major limitation high battery consumption. Therefore an upgrade version of this technology with low energy consumption was introduced, called BLE (Bluetooth Low Energy).
Android 4.3 (API level 18) introduces built-in platform support for Bluetooth Low Energy (BLE) in the central role and provides APIs that apps can use to discover devices, query for services, and transmit information. (Android Developers Doc)
This allows Android applications to communicate with BLE devices that have stricter power requirements, such as proximity sensors, heart rate monitors, fitness devices, home Automation System, Medical services and the Automotive industry.
BLE Concepts:
These are the few concepts to understand in BLE which is used to easily communicate with other devices.
GATT Profile
GATT stands for Generic Attribute Profile. All devices implement one or more Profile.The profile is a specification for how a device works in a particular application.GATT is a general specification for sending and receiving short pieces of data (known as attributes) over the low energy link.
Attribute Protocol
GATT is built on top of the Attribute Protocol. This is also referred to as GATT/ATT. Attribute Protocol (ATT) is optimized to run on BLE devices. To this end, it uses as few bytes as possible. Each attribute is uniquely identified by a Universally Unique Identifier (UUID). Which is the standardized 128-bit format for a string ID used to uniquely identify information. The attribute transported by ATT formatted as characteristics and service.
Characteristic
A Characteristic is a data value transferred between client and server. A characteristic contains a single value and 0-n describes the characteristic value. A characteristic can be thought as a type, analogous to a class.
Descriptor
A service is a group of characteristics that operate together to perform a specific function. Many devices implement the Device Information Service. This service is made up of characteristics such as manufacturer name, model name, and firmware revision.
Implementation of Bluetooth Low Energy (BLE):
First of all, create a new project in the android studio.
File – > New – > New Project.
Please select the API level 18 Because it can not used old device due to change of the Bluetooth specification.Please specify the SDK version 18 in your app.
Bluetooth Low Energy (BLE) permission in App:
If you want to use, manipulate and discover the Bluetooth setting you must also declare the BLUETOOTH_ADMIN permission in you project manifest file.You must include the BLUETOOTH permission in your manifest file due to use permission BLUETOOTH_ADMIN.
If your app contains the BLE features than declaring the below permission in manifest file.
You can check device is supported the BLE or not. Then you can disable the related feature in the app.
If(! getPackageManager().hasSystemFeature(PackageManager.FEATURE_BLUETOOTH_LE))
{
Toast.makeText(this, “BLUETOOTH is not supported in you device”, Toast.LENGTH_SHORT).show();
finish();
}
Get the BluetoothAdapter:
Bluetooth Adapter is required for any and all activity related to BLUETOOTH. Initializes a Bluetooth adapter get the reference to BluetoothAdapter through BluetoothManager.
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter mBluetoothAdapter = bluetoothManager.getAdapter();
Check Bluetooth is enabled or not
If you need to check the Bluetooth is enabled or not you can use isEnabled(). If it returns the false , then Bluetooth is disabled. Otherwise, you enable the Bluetooth in the device
if(mBluetoothAdapter == null || !mBluetoothAdapter.isEnabled())
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
To Find the Bluetooth Devices:
To find BLE devices, you use the startLeScan() method. This method takes BluetoothAdapter.LeScanCallback as a parameter. You must implement this callback, because that is how scan results are returned.
startLeScan()
private void scanLeDevice(final boolean enable) {
if (enable) {
// Stops scanning after a pre-defined scan period.
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
mScanning = false;
mBluetoothAdapter.stopLeScan(mLeScanCallback);
}
}, SCAN_PERIOD);
mScanning = true;
mBluetoothAdapter.startLeScan(mLeScanCallback);
} else {
mScanning = false;
mBluetoothAdapter.stopLeScan(mLeScanCallback);
}
}
This is LeScanCallback which is return the list of device.
private BluetoothAdapter.LeScanCallback mLeScanCallback =
new BluetoothAdapter.LeScanCallback() {
@Override
public void onLeScan(final BluetoothDevice device, int rssi,
byte[] scanRecord) {
runOnUiThread(new Runnable() {
@Override
public void run() {
mLeDeviceListAdapter.addDevice(device);
mLeDeviceListAdapter.notifyDataSetChanged();
}
});
}
};
Connecting to a GATT Server
Every BLE device has owned GATT profile through which they can act as a server. Like all network devices, Bluetooth LE also works in a client/server manner. The above step is used for getting a list of BLE enabled devices. now we want to connect other device using the GATT server. To connect to a GATT server on a BLE device, you use the connectGatt() method. This method takes three parameters: a Context object, autoConnect (boolean indicating whether to automatically connect to the BLE device as soon as it becomes available), and a reference to a BluetoothGattCallback.
BluetoothGatt mBluetoothGatt = device.connectGatt(this, false, mGattCallback);
This BluetoothGattCallback connects to the GATT server hosted by the BLE device, and returns a BluetoothGatt instance, which you can then use to conduct GATT client operations. The caller (the Android app) is the GATT client. The BluetoothGattCallback is used to deliver results to the client, such as connection status, as well as any further GATT client operations.
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState)
{
Log.i("onConnectionStateChange", "Status: " + status);
if(newState == BluetoothProfile.STATE_CONNECTED)
{
Log.i("gattCallback", "STATE_CONNECTED");
gatt.discoverServices();
}
if(newState == BluetoothProfile.STATE_DISCONNECTED)
{
BluetoothProfile.STATE_DISCONNECTED:
Log.e("gattCallback", "STATE_DISCONNECTED");
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
List services = gatt.getServices();
Log.i("onServicesDiscovered", services.toString());
gatt.readCharacteristic(services.get(1).getCharacteristics().get
(0));
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt,
BluetoothGattCharacteristic
characteristic, int status) {
Log.i("onCharacteristicRead", characteristic.toString());
gatt.disconnect();
}
};
The BluetoothGattCallback containsonConnectionStateChange, onServicesDiscovered,onCharacteristicRead methods.
onConnectionStateChange:
Callback indicating when a remote device has been connected or disconnected.
onServicesDiscovered:
Callback invoked when the list of remote services, characteristics and descriptors for the remote device have been updated, ie new services have been discovered.
onCharacteristicRead:
Callback reporting the result of a characteristic read operation.
Conclusion
The Above example is use for scanning list of BLE (Bluetooth Low Energy) devices and discover their services and read the basic characteristics of it. BLE which is used to easily communicate with other devices. BLE ( Bluetooth Low Energy ) take very low power Consumption So Now a days Bluetooth Low Energy is using hug Android devices, Home Automation System, Medical services and Automotive industry.